Hi, I seem a little lost on this, what I want to do is make the forward and back buttons in my webView gray out if there is no browsing activity, just like in Safari. Screenshot and code:
Web.h:
and
Web.m:
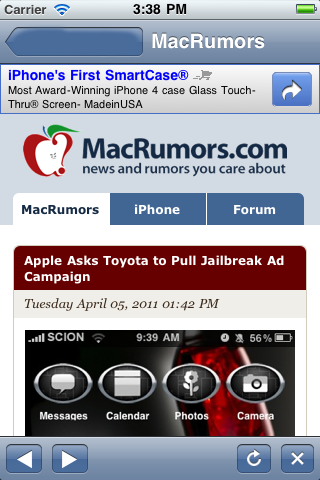
Web.h:
Code:
#import <UIKit/UIKit.h>
@interface Web: UIViewController {
UIWebView *webView;
UIActivityIndicatorView *activityIndicator;
NSTimer *timer;
}
@property (nonatomic, retain) IBOutlet UIWebView *webView;
@property (nonatomic, retain) IBOutlet UIActivityIndicatorView *activityIndicator;
@property (nonatomic, retain) NSTimer *timer;
@end
Web.m:
Code:
#import "Web.h"
@implementation Web
@synthesize webView;
@synthesize activityIndicator;
@synthesize timer;
- (BOOL)shouldAutorotateToInterfaceOrientation:
(UIInterfaceOrientation)interfaceOrientation {
return (interfaceOrientation !=
UIInterfaceOrientationPortraitUpsideDown);
}
// Implement viewDidLoad to do additional setup after loading the view, typically from a nib.
- (void)viewDidLoad {
[super viewDidLoad];
[webView addSubview: activityIndicator];
self.title = @"MacRumors";
NSString *urlAddress = @"https://www.macrumors.com/";
NSURL *url = [NSURL URLWithString:urlAddress];
NSURLRequest *requestObj = [NSURLRequest requestWithURL:url];
[webView loadRequest:requestObj];
timer = [NSTimer scheduledTimerWithTimeInterval:(1.0/2.0) target:self selector:@selector(loading) userInfo:nil repeats:YES];
}
-(void)loading{
if(!webView.loading)
[activityIndicator stopAnimating];
else
[activityIndicator startAnimating];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning]; // Releases the view if it doesn't have a superview
// Release anything that's not essential, such as cached data
}
- (void)dealloc {
[webView release];
[activityIndicator release];
[timer release];
[super dealloc];
}
@end